5 Software Development Principles That Transformed My Coding Career
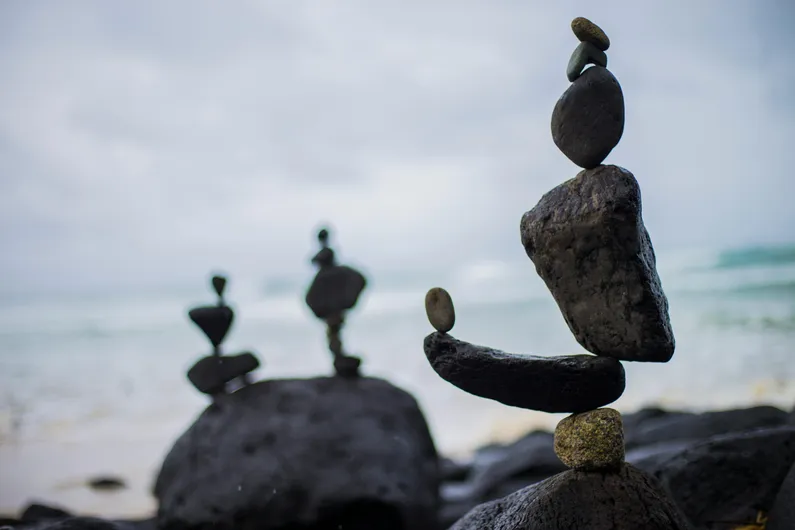
In the ever-evolving world of software development, certain principles stand the test of time. These aren’t just abstract concepts—they’re practical approaches that have saved me countless hours of debugging and made my code more maintainable, flexible, and just plain better. Let’s dive into the game-changing principles that have transformed my journey as a developer.
The Inversion of Control: Building Extensible Software
Have you ever found yourself trapped by your own code? I certainly have. That’s where Inversion of Control (IoC) comes in to save the day.
IoC flips the traditional control flow on its head. Instead of your code making all the decisions, you create flexible frameworks where components receive their dependencies rather than creating them. This shift in mindset leads to more modular, testable, and extensible code.
“The key benefit of IoC is that it decouples the execution of a task from implementation.”
In practice, this might look like:
- Dependency injection to provide objects their dependencies
- Event-driven programming where execution depends on events
- Template method patterns that allow child classes to extend behavior
By implementing IoC, I’ve built systems that can grow and adapt without requiring rewrites of core functionality—a true game-changer when working on long-lived projects.
Embracing Composability: Building Lego-Like Code
Composability has revolutionized how I approach software design. Think of your code components as Lego bricks—each piece should be self-contained but designed to snap together with others to build something greater.
Why Composability Matters:
- It promotes code reuse
- It simplifies testing (test small pieces individually)
- It makes your codebase more understandable
When functions and components do one thing well and can be mixed and matched, magic happens. Your system becomes more flexible and adaptation to changing requirements becomes significantly easier.
I’ve found that adopting functional programming concepts—pure functions, immutability, and function composition—has dramatically improved my ability to write composable code.
The AHA Programming Principle: Avoid Hasty Abstractions
Kent C. Dodds introduced me to the AHA principle (Avoid Hasty Abstractions), and it completely changed my approach to abstractions.
In his influential article “AHA Programming”, Dodds challenges the DRY (Don’t Repeat Yourself) principle that many of us hold sacred. He suggests that sometimes, a little duplication is better than the wrong abstraction.
Premature abstraction can lead to:
- Overly complex code that’s hard to modify
- Rigid systems that resist change
- Cognitive overhead for team members
The AHA principle encourages us to:
- Wait until we see patterns emerge naturally
- Prefer duplication over the wrong abstraction
- Make abstractions only when the benefits are clear and concrete
Following this principle has helped me create more intuitive and maintainable codebases. I’ve learned to be patient and let the right abstractions reveal themselves through use cases rather than trying to predict them upfront.
The Boy Scout Rule: Always Leave Code Better Than You Found It
What if every developer made just a small improvement each time they touched a codebase? This simple idea forms the basis of The Boy Scout Rule in programming.
Inspired by the Boy Scouts’ rule to “leave the campground cleaner than you found it,” this principle encourages developers to make small improvements to code whenever they work on it.
These improvements might include:
- Clarifying variable names
- Adding missing documentation
- Refactoring confusing logic
- Removing dead code
- Adding or improving tests
The beauty of this approach is that it doesn’t require massive refactoring efforts. Small, incremental improvements compound over time, gradually elevating the quality of the entire codebase.
I’ve seen firsthand how teams that adopt this principle create an upward quality spiral—technical debt decreases rather than accumulates, and development velocity increases over time.
Getting to the Root: Solving Problems at Their Source
The final principle that transformed my coding career is what I call “Root Cause Resolution.” It’s about having the discipline to dig deeper when bugs appear instead of applying quick fixes.
The Problem with Quick Fixes:
Have you ever fixed a bug only to see it reappear in a slightly different form? I’ve been there too many times. Quick fixes often treat symptoms rather than underlying causes, creating:
- Endless loops of similar bugs
- Growing technical debt
- Increasing system fragility
- Developer frustration
When a bug surfaces, I now ask:
- “Why did this happen in the first place?”
- “What underlying design issue allowed this bug to exist?”
- “How can I prevent this entire class of bugs?”
This approach takes more time initially but pays massive dividends. By addressing root causes, I’ve reduced bug recurrence rates and built more robust systems.
Bringing It All Together
These principles aren’t just theoretical—they’re practical approaches that have genuinely transformed my effectiveness as a developer:
Inversion of Control : Creates extensible, adaptable systems
Composability : Enables flexible, reusable code
AHA Programming : Prevents premature abstraction pitfalls
Boy Scout Rule : Gradually improves codebase quality
Root Cause Resolution : Prevents recurring bugs
By incorporating these principles into your daily coding practice, you’ll not only write better code but also become a more valuable developer to any team.
What principles have transformed your coding journey? I’d love to hear about your experiences in the comments below!
About the Author: A passionate software developer with over a decade of experience in building scalable, maintainable systems. Constantly learning and sharing insights from the trenches of professional software development.
Keywords: software development principles, inversion of control, composability, AHA programming principle, Boy Scout Rule, root cause analysis, code quality, software engineering best practices, Kent C Dodds